Jak zaktualizować stan rodzica z komponentu dziecka w React Native ?
Przekazywanie danych z komponentu dziecka do komponentu rodzica jest łatwe. Wystarczy, że umieszczając komponent dziecko w treści rodzica przekażemy do niego funkcję, która ustawia w stanie komponentu rodzica odpowiednie dane, a następnie uruchomimy te dane z komponentu dziecka, np. poprzez kliknięcie w przycisk. Schemat przedstawia się następująco:
Parent.js
import React, {Component} from 'react';
import {StyleSheet, Text, View, TouchableHighlight, Image} from 'react-native';
import Child from './Child';
export default class Parent extends Component {
constructor(props) {
super(props);
this.state = {
data: ''
};
}
passData = (passedData) => {
this.setState({ data: passedData });
};
render() {
return (
<View>
<Child passData={this.passData} />
<Text> {this.state.data} </Text>
</View>
);
}
}
Child.js
import React, {Component} from 'react';
import {Text, View, TouchableHighlight,} from 'react-native';
export default class Child extends Component {
constructor(props) {
super(props);
this.state = {
data: "Yeah, it's working!"
};
}
render() {
return (
<View>
<TouchableHighlight onPress={() => this.props.passData(this.state.data)} >
<Text style={{fontSize: 20, backgroundColor: 'yellow', padding: 20}}> Pass data to parent </Text>
</TouchableHighlight>
</View>
);
}
}
Efekt będzie jak poniżej:
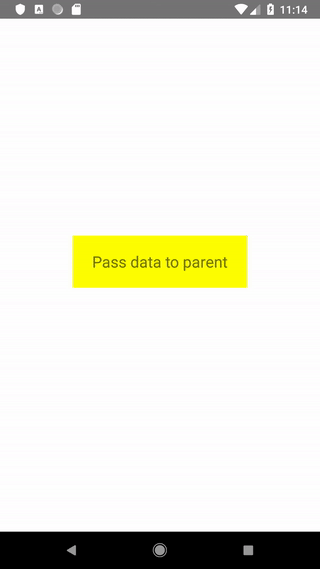