Ok, we want to display a list of items in React Native. We can do it in several ways, but here – two will be presented – ScrollView and FlatList.
ScrollView is a component that should have a limited height (set e.g. by setting the {flex: 1} parameter). It renders all the children at once, so if there are a lot of them, the application loads slowly. This can create performance issues because the application must wait to render all children components before displaying them on the screen. The ScrollView component is therefore suitable for smaller lists.
To rendering larger lists is better to use FlatList component, which loads only some of children components (so-called lazy loading), especially those that are currently visible on the screen. When scrolling, FlatList loads new components and removes those that are no longer visible. In addition, it offers a number of other functionalities that ScrollView, does not offer, you can find a list here: Flatlist
Let’s create a new folder called: components, and two components in it: the first will contain our list: List.js, and the second will contain data that the list should display: Data.js.
The App.js component should return our main component with a list, so the code will be very simple:
App.js:
import React, {Component} from 'react';
import List from './components/List';
export default class App extends Component {
render() {
return <List />
}};
However, our data will look like this:
Data.js:
export default Data = [
{id: 1, text: 'Item One', color: 'red'},
{id: 2, text: 'Item Two', color: 'blue'},
{id: 3, text: 'Item Three', color: 'yellow'},
{id: 4, text: 'Item Four', color: 'green'},
{id: 5, text: 'Item Five', color: 'orange'},
{id: 6, text: 'Item Six', color: 'red'},
{id: 7, text: 'Item Seven', color: 'blue'},
{id: 8, text: 'Item Eight', color: 'yellow'},
{id: 9, text: 'Item Nine', color: 'green'},
{id: 10, text: 'Item Ten', color: 'orange'},
{id: 11, text: 'Item Eleven', color: 'red'},
{id: 12, text: 'Item Twelve', color: 'blue'},
{id: 13, text: 'Item Thirteen', color: 'yellow'},
{id: 14, text: 'Item Fourteen', color: 'green'},
{id: 15, text: 'Item Fifteen', color: 'orange'},
]
React Native ScrollView Example
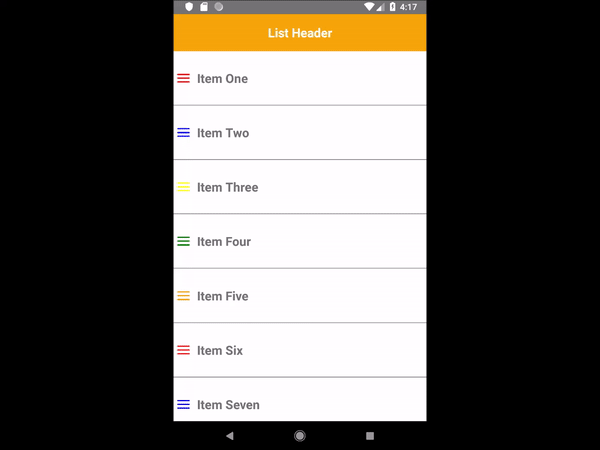
We’ll start with the List.js component. To display data in a list using ScrollView, we need to import several components from react-native. and render them on the screen:
List.js:
import React, {Component} from 'react';
import {StyleSheet, Text, View, ScrollView } from 'react-native';
import Data from './Data';
export default class List extends Component {
render() {
return (
<View style={styles.contentContainer}>
<View style={styles.header}>
<Text style={styles.headerText}> List Header </Text>
</View>
<ScrollView >
<View>
{item}
</View>
</ScrollView>
</View>
)
}
};
Above, we return the header containing the text, and below it is the ScrollView component, containing children components that we can scroll. We will return the child components from the Data.js component
const item = Data.map( (item, index) => {
return <View key={item.id} style={styles.item}>
<View style={styles.marginLeft}>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
</View>
<Text style={styles.text}>{item.text} </Text>
</View>
})
Finally, we will add styles:
const styles = StyleSheet.create({
header: {
height: 60,
backgroundColor: 'orange',
alignItems: 'center',
justifyContent: 'center',
},
headerText: {
fontSize: 20,
fontWeight: 'bold',
color: 'white',
},
contentContainer: {
backgroundColor: 'white',
},
item: {
flexDirection: 'row',
borderBottomWidth: 1,
borderBottomColor: 'grey',
alignItems: 'center',
},
marginLeft: {
marginLeft: 5,
},
menu: {
width: 20,
height: 2,
backgroundColor: '#111',
margin: 2,
borderRadius: 3,
},
text: {
marginVertical: 30,
fontSize: 20,
fontWeight: 'bold',
marginLeft: 10
, }
})
The whole thing will look like this:
List.js:
import React, {Component} from 'react';
import {StyleSheet, Text, View, ScrollView } from 'react-native';
import Data from './Data';
export default class List extends Component {
render() {
const item = Data.map( (item, index) => {
return <View key={item.id} style={styles.item}>
<View style={styles.marginLeft}>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
</View>
<Text style={styles.text}>{item.text} </Text>
</View>
})
return (
<View style={styles.contentContainer}>
<View style={styles.header}>
<Text style={styles.headerText}> List Header </Text>
</View>
<ScrollView >
<View>
{item}
</View>
</ScrollView>
</View>
)
}
};
const styles = StyleSheet.create({
header: {
height: 60,
backgroundColor: 'orange',
alignItems: 'center',
justifyContent: 'center',
},
headerText: {
fontSize: 20,
fontWeight: 'bold',
color: 'white',
},
contentContainer: {
backgroundColor: 'white',
},
item: {
flexDirection: 'row',
borderBottomWidth: 1,
borderBottomColor: 'grey',
alignItems: 'center',
},
marginLeft: {
marginLeft: 5,
},
menu: {
width: 20,
height: 2,
backgroundColor: '#111',
margin: 2,
borderRadius: 3,
},
text: {
marginVertical: 30,
fontSize: 20,
fontWeight: 'bold',
marginLeft: 10
, }
})
React Native FlatList Example
Czas na kolejną listę. Tym razem zamiast ScrollView użyjemy komponentu Flatlist. W tym wypadku nie ma potrzeby samodzielnego mapowania danych, flatlista zrobi to za nas. Dane odczytane zostaną z właściwości data. Podobnie jak w przypadku ScrollView, we flatliście każde dziecko powinno być oznaczone innym kluczem. Możemy w tym celu użyć właściwości keyExtractor, która przyporządkuje odpowiedni klucz do dziecka. Klucz ten powinien być podany w stringu. Funkcja renderItem wyrenderuje komponenty-dzieci według podanego przez nas schematu. Komponent List.js będzie zatem wyglądał tak:
This time we will use the Flatlist component instead of ScrollView. In this case, there is no need for individual data mapping, the flatlist will do it for us. The data will be read from the data property. As with ScrollView, each child should be marked with a different key in the flatlist. We can use the keyExtractor property, which assigns the appropriate key to the child. This key should be given in the string. The renderItem function will render child components according to the scheme given by us. The List.js component will be look like this:
List.js
import React, {Component} from 'react';
import {StyleSheet, Text, View, FlatList } from 'react-native';
import Data from './Data';
export default class List extends Component {
renderItem = ({item}) => (
<View style={styles.item}>
<View style={styles.marginLeft}>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
<View style={[styles.menu, { backgroundColor: item.color }]}></View>
</View>
<Text style={styles.text}> {item.text} </Text>
</View>
)
render() {
return (
<View style={styles.contentContainer}>
<View style={styles.header}>
<Text style={styles.headerText}> List Header </Text>
</View>
<FlatList
data={Data}
keyExtractor={(item) => item.id.toString()}
renderItem={this.renderItem}
/>
</View>
)
}
};
const styles = StyleSheet.create({
header: {
height: 60,
backgroundColor: 'orange',
alignItems: 'center',
justifyContent: 'center',
},
headerText: {
fontSize: 20,
fontWeight: 'bold',
color: 'white',
},
contentContainer: {
backgroundColor: 'white',
},
item: {
flexDirection: 'row',
borderBottomWidth: 1,
borderBottomColor: 'grey',
alignItems: 'center',
},
marginLeft: {
marginLeft: 5,
},
menu: {
width: 20,
height: 2,
backgroundColor: '#111',
margin: 2,
borderRadius: 3,
},
text: {
marginVertical: 30,
fontSize: 20,
fontWeight: 'bold',
marginLeft: 10
, }
})
The effect is similar to that in the previous case, but the functionality is a little different (as I mentioned at the beginning), just to remind you – the Flatlist does not render all components-children at once, and ScrollView does.
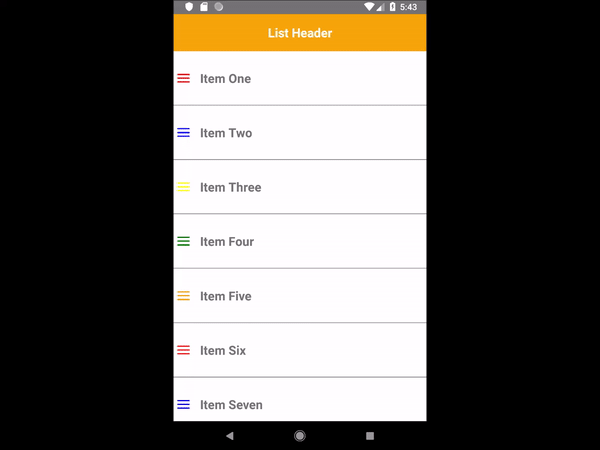