Jak wiemy z dokumentacji React Native, ScrollView renderuje wszystkie swoje dzieci na raz, co przy dużej ich ilości może powodować problemy z wydajnością. Istnieje jednak sposób, by rozłożyć renderowanie dużej ilości danych w czasie tak, aby użytkownik nie odczuł tych problemów. Możemy wyświetlić przykładowo 20 elementów przy pierwszym wczytaniu danych i dodawać kolejno nowe elementy w miarę przewijania listy. Tak stworzymy “niekończące się” przewijanie, tzw. “infnite scroll”.
As we know from React Native documentation, ScrollView renders all of its children at once, which can cause performance problems with a large number of them. However, there is a way to spread in time rendering of large number of data, so that the user does not feel these problems. For example, we can display 20 items at the first time when load data and add new items one by one while scrolling the list. That’s how we’ll create “endless” scrolling, so-called “infnite scroll”.
Implementing infinite scrolling on React Native’s scrollview
In this post: https://love-coding.pl/en/react-native-rendering-lists-with-scrollview-and-flatlist/ we created a list of elements with ScrollView. Now we need to add a condition to it, that will display the right number of tasks on the screen. Let’s start with determining the initial number of rendered tasks, let it be 20. So in the component state of the itemToRender value we assign the number 20.
this.state = {
itemToRender: 20,
data: this.data,
}
Then, displaying each element of the list depends on the condition:
const item = this.state.data.map( (item, index) => {
if (index+1 <= this.state.itemToRender) {
return ...
}
})
Finally, in ScrollView we will add a condition, after which the number of displayed data will increase. Let this condition be that the scroll bar reaches the list height minus 50. However, how do we know that the list has been scrolled completely? We need to write our own check condition, something like “scroll to bottom”.
<ScrollView
contentContainerStyle={{paddingBottom: 70}}
onMomentumScrollEnd={(e) => {
const scrollPosition = e.nativeEvent.contentOffset.y;
const scrolViewHeight = e.nativeEvent.layoutMeasurement.height;
const contentHeight = e.nativeEvent.contentSize.height;
const isScrolledToBottom = scrolViewHeight + scrollPosition
// check if scrollView is scrolled to bottom and limit itemToRender to data length
if (isScrolledToBottom >= (contentHeight-50) && this.state.itemToRender <= this.state.data.length ) {
this.setState({ itemToRender: this.state.itemToRender + 20 })
}
}}
>
Now, after turning on our application, it will render only 20 tasks (which will significantly reduce data loading time), and will render next tasks only after scrolling the scroll bar almost to the end. Effect:
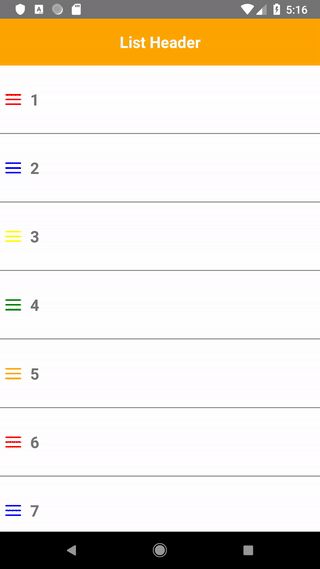