If you have problem with understanding how Drawer navigation works, this short tutorial will help you implement DrawerNavigator into your application
If you don’t have navigation installed in your project yet, you can do it by entering the commands:
npm install --save react-navigation
npm install --save react-native-gesture-handler
react-native link react-native-gesture-handler
Let’s start from creating components folder, in which we put DrawerNavigator.js, HomeScreen.js, Screen1.js, Screen2.js, Screen3.js files.
App.js file looks like this:
import React, {Component} from 'react';
import DrawerNavigator from './components/DrawerNavigator';
export default class App extends Component {
render() {
return (
<DrawerNavigator />
);
}
}
It’s time to create DrawerNavigator:
DrawerNavigator.js
import React, {Component} from 'react';
import { createDrawerNavigator, createAppContainer} from 'react-navigation';
import HomeScreen from './HomeScreen';
import Screen1 from './Screen1';
import Screen2 from './Screen2';
import Screen3 from './Screen3';
const MyDrawerNavigator = createDrawerNavigator({
Home: {screen: HomeScreen},
Screen1: {screen: Screen1},
Screen2: {screen: Screen2},
Screen3: {screen: Screen3},
},
{
initialRouteName: 'Home',
drawerWidth: 300,
drawerPosition: 'left',
}
);
const AppContainer = createAppContainer(MyDrawerNavigator);
export default class DrawerNavigator extends Component {
render() {
return <AppContainer />;
}
}
We assign a screen with the appropriate name to each of the 4 navigator options. As default, we set the screen assigned to the Home name, assigning it to initialRouteName. We set the navigator width to 300 and set it on the left side of the main screen.
On the main screen, we put text: This is the start screen and the icon, after touching which our navigator will slide out on the left.
HomeScreen.js
import React, {Component} from 'react';
import {StyleSheet, Text, View, TouchableHighlight} from 'react-native';
import { DrawerActions, } from 'react-navigation';
export default class HomeScreen extends Component {
static navigationOptions = {
drawerLabel: 'Home',
};
render() {
return (
<View style={styles.view}>
<TouchableHighlight style={styles.touchableHighlight} underlayColor={'rgba(0,0,0,0.8)'}
onPress={() => { this.props.navigation.dispatch(DrawerActions.openDrawer()); }}>
<Text style={styles.open}>OPEN </Text>
</TouchableHighlight>
<Text style={styles.text}> This is homescreen </Text>
</View>
);
}
}
Other screens will have different colors and a button that redirect to the main screen:
Screen1.js, Screen2.js Scree3.js
import React, {Component} from 'react';
import {StyleSheet, Text, View, TouchableHighlight} from 'react-native';
export default class Screen1 extends Component {
render() {
return (
<View style={styles.view}>
<Text style={styles.text}> This is screen 1 </Text>
<TouchableHighlight onPress={() => this.props.navigation.goBack() } style={styles.touchableHighlight} underlayColor={'#f1f1f1'}>
<Text style={styles.text}>Go back</Text>
</TouchableHighlight>
</View>
);
}
}
Now we must set styles:
HomeScreen,js
const styles = StyleSheet.create({
view: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: 'white',
},
text: {
fontSize: 26,
color: 'purple'
},
touchableHighlight: {
width: 50,
height: 50,
backgroundColor: 'red',
borderRadius: 50,
alignItems: 'center',
justifyContent: 'center',
position: 'absolute',
left: 10,
top: 10,
},
open: {
color: 'white',
fontSize: 16,
fontWeight: 'bold',
},
});
Screen1.js
const styles = StyleSheet.create({
view: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: 'yellow',
},
text: {
fontSize: 20,
color: 'black',
},
touchableHighlight: {
backgroundColor: 'orange',
paddingVertical: 20,
paddingHorizontal: 50,
margin: 10,
}
});
The effect is:
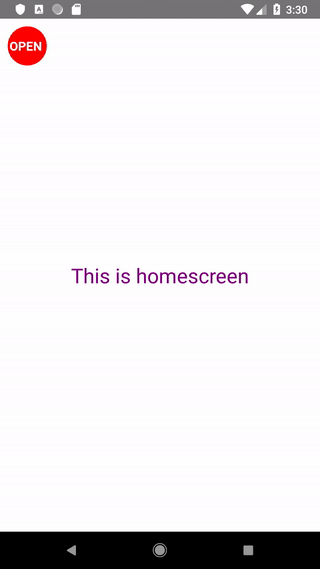
Customize DrawerNavigator
And what if we would like to add more elements to our navigator? We could use a headline, a picture or a footer. In this case, we can set our own personalized component that will serve as DrawerNavigator. To do that, we will slightly modify our navigator:
DrawerNavigator.js
...
{
initialRouteName: 'Home',
contentComponent: ContentComponent,
drawerWidth: Dimensions.get('window').width,
drawerPosition: 'left',
drawerBackgroundColor: 'transparent',
}
...
Now we can create any look by adding e.g. a header with a photo and links to other screens, as well as appropriate icons. In fact, we can add what we want here, which makes our navigator really interesting. Sample code below:
ContentComponent.js
export default class ContentContainer extends Component {
render() {
return (
<TouchableOpacity activeOpacity={1} style={styles.drawerTransparent} onPress={() => this.props.navigation.goBack()}>
<TouchableOpacity activeOpacity={1} style={styles.drawer} disabled={false}>
<ScrollView>
<View style={styles.header}>
<Image source={require('../face4.jpg')} style={styles.headerImage}/>
<Text style={[styles.text, {color: 'white'}]}>My Profile</Text>
</View>
<TouchableHighlight underlayColor={'rgba(0,0,0,0.2)'} onPress={() => this.props.navigation.navigate('Screen1')}>
<View style={styles.row}>
<Image source={require('../icons/contact.jpg')} style={styles.headerImage} />
<Text style={styles.text}>Contacts</Text>
</View>
</TouchableHighlight>
<TouchableHighlight underlayColor={'rgba(0,0,0,0.2)'} onPress={() => this.props.navigation.navigate('Screen2')}>
<View style={styles.row}>
<Image source={require('../icons/user.jpg')} style={styles.headerImage} />
<Text style={styles.text}>Add Contact</Text>
</View>
</TouchableHighlight>
<TouchableHighlight underlayColor={'rgba(0,0,0,0.2)'} onPress={() => this.props.navigation.navigate('Screen3')}>
<View style={styles.row}>
<Image source={require('../icons/group.jpg')} style={styles.headerImage} />
<Text style={styles.text}>Add Group</Text>
</View>
</TouchableHighlight>
<View style={styles.line}></View>
...
</View>
</TouchableHighlight>
</ScrollView>
</TouchableOpacity>
</TouchableOpacity>
);
}
}
Efect:
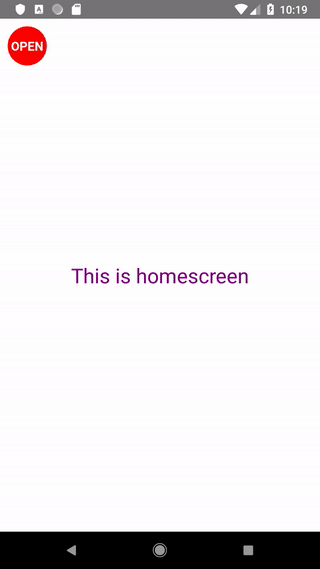
while clicking the background of screen the menu still not closed.Please help to solve