It often happens that we want to change the dimensions of the button, which by default is extended to the entire width of the parent element. While reducing its width is not a problem – it is enough to reduce the width of the parent, but changing the height is already problematic. The Button element has no style property, so apart from changing the text color on iOS and the background color on Android, we can not set much in it.
To have more control over the button, it is better to use TouchableOpacity or TouchableNativeFeedback instead.
There is comparison of Button and TouchableOpacity below:
import React, {Component} from 'react';
import {StyleSheet, View, Text, Button, TouchableOpacity } from 'react-native';
export default class DimensionsComponent extends Component {
render() {
return (
<View style={styles.parent}>
<Button title='Button' />
<TouchableOpacity activeOpacity={0.95} style={styles.button}>
<Text style={styles.text}>Button</Text>
</TouchableOpacity>
</View>
)
}
}
const styles = StyleSheet.create({
parent: {
width: 300,
height: 500,
backgroundColor: 'red',
margin: 50,
},
button: {
flexDirection: 'row',
height: 50,
backgroundColor: 'yellow',
alignItems: 'center',
justifyContent: 'center',
marginTop: 50,
elevation:3,
},
text: {
fontSize: 16,
fontWeight: 'bold',
}
})
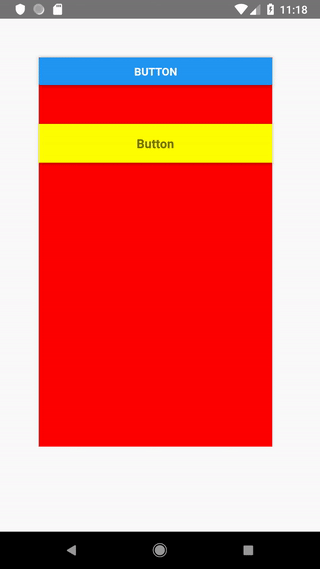
If you want to set the button to the entire width of the screen, it would be best if the parent element also occupied the entire width.
parent: {
flex: 1,
backgroundColor: 'red',
},
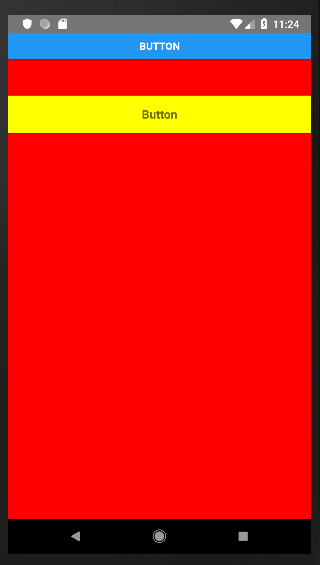