How to update parent state from child component in React Native ?
Passing data from child component to parent component is easy. When we place child component in parent’s content we must use function (sets in parent component and invoked in child component), which sets data in the parent’s component state, when action is made in child’s component, e.g. by clicking the button. The diagram is as follows:
Parent.js
import React, {Component} from 'react';
import {StyleSheet, Text, View, TouchableHighlight, Image} from 'react-native';
import Child from './Child';
export default class Parent extends Component {
constructor(props) {
super(props);
this.state = {
data: ''
};
}
passData = (passedData) => {
this.setState({ data: passedData });
};
render() {
return (
<View>
<Child passData={this.passData} />
<Text> {this.state.data} </Text>
</View>
);
}
}
Child.js
import React, {Component} from 'react';
import {Text, View, TouchableHighlight,} from 'react-native';
export default class Child extends Component {
constructor(props) {
super(props);
this.state = {
data: "Yeah, it's working!"
};
}
render() {
return (
<View>
<TouchableHighlight onPress={() => this.props.passData(this.state.data)} >
<Text style={{fontSize: 20, backgroundColor: 'yellow', padding: 20}}> Pass data to parent </Text>
</TouchableHighlight>
</View>
);
}
}
Effect is visible below:
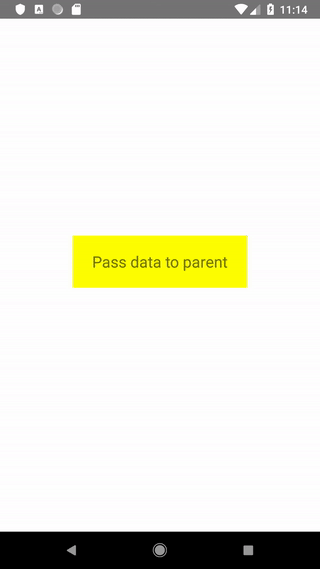