The problem of centering divs or text is quite common and I often had to spend more time fixing it than I would like. Sometimes the situation is simple and using one of the basic methods, like margin: 0 auto solves the case, and sometimes you have to think a little more. Therefore, below are some useful methods that you can use depending on your needs.
Centering div
1. margin: 0 auto;
We can use this option if the element you want to center is a block element and has defined width. In this way, we center the div or paragraph vertically.
Let’s create two divs, one is larger and contains the second, smaller. We center both vertically using margin: 0 auto;
HTML:
<body>
<div class="outside">
<div class="inside">
</div>
<p class="paragraph"> Centered paragraph </p>
</div>
</body>
CSS:
.outside {
width: 600px;
height: 400px;
border: 2px solid black;
margin: 0 auto;
}
.inside {
width: 150px;
height: 100px;
background-color: red;
margin: 0 auto;
}
.paragraph {
width: 150px;
margin: 0 auto;
}
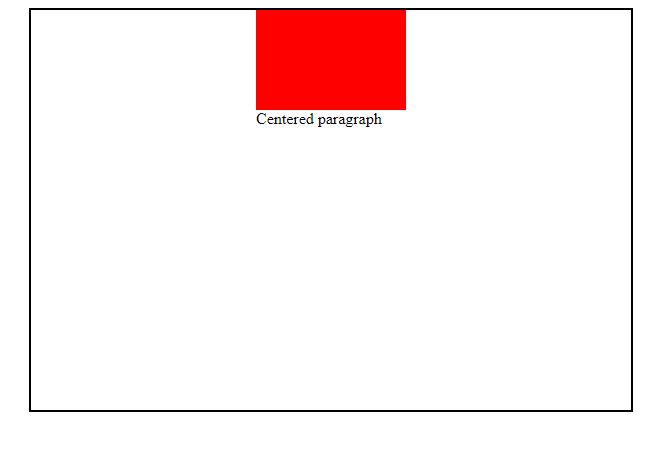
2. Flexbox
Za pomocą flexboxa możemy wycentować jednego lub kilka div-ów zarówno w poziomie jak i w pionie. Wystarczy, że element – rodzic posiadać będzie właściwość display: flex i że ustawimy na min odpowiednie zachowanie elementów – dzieci.
Using a flexbox, we can center one or several divs both – horizontally and vertically. It’s enough that the parent element has the display property set to: flex and that we set the appropriate behavior of children elements.
HTML:
<body>
<div class="outside">
<div class="inside">
</div>
</div>
</body>
CSS:
.outside {
width: 600px;
height: 400px;
border: 2px solid black;
display: flex;
justify-content: center;
align-items: center;
}
.inside {
width: 150px;
height: 100px;
background-color: red;
}
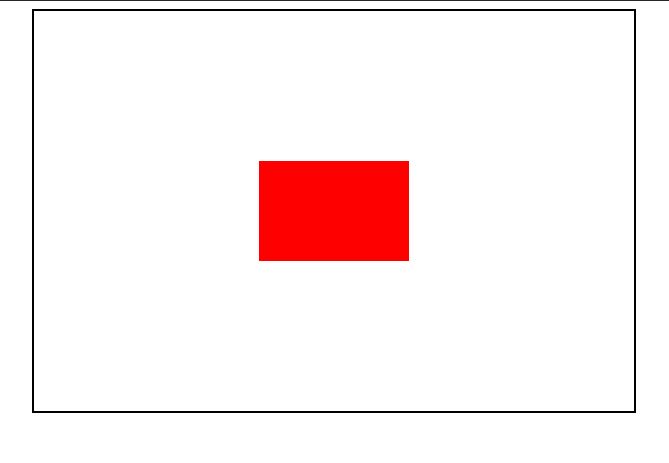
3. CSS Transform
It is less common technics, but we can use it as so:
HTML:
<body>
<div class="outside">
<div class="inside"> </div>
</div>
</body>
CSS:
.outside {
width: 600px;
height: 400px;
border: 2px solid black;
position: relative
}
.inside {
width: 150px;
height: 100px;
background-color: red;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
Effect is the same as in flexbox:
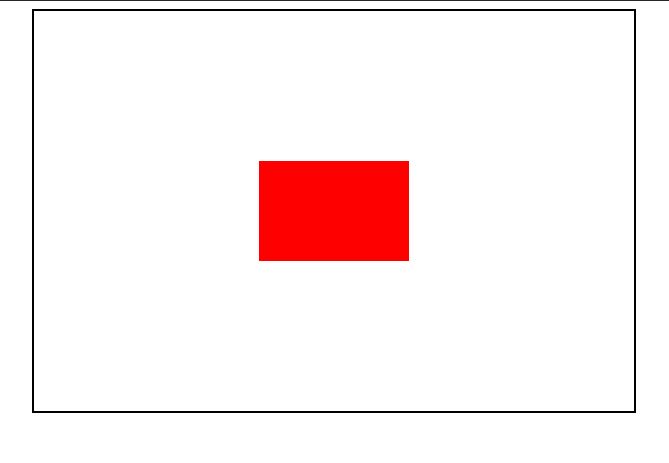
4. Position: absolute
In this case we have child that has absolute positioning, margins setted to auto and from each side we set 0 pixels, so child is centered in parent element.
HTML:
<body>
<div class="outside">
<div class="inside"> </div>
</div>
</body>
CSS:
.outside {
width: 600px;
height: 400px;
border: 2px solid black;
margin: 0 auto;
position: relative;
}
.inside {
width: 150px;
height: 100px;
background-color: red;
position: absolute;
margin: auto;
top: 0;
right: 0;
bottom: 0;
left: 0;
}
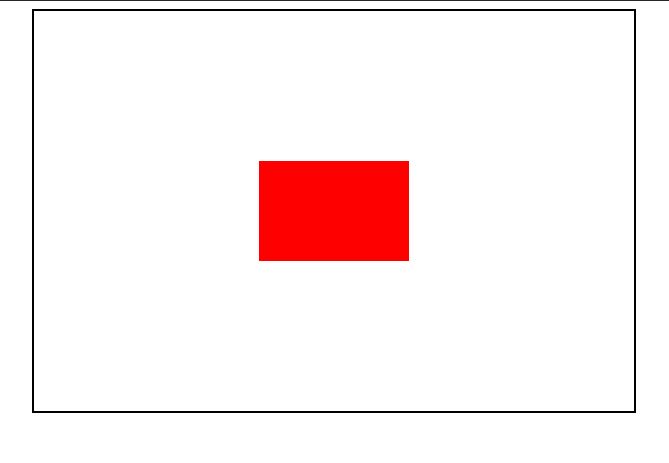
Text centering
1. Text-align
When it comes to text vertically centering, one of the best-known rules is to use the text-align property.
HTML:
<p> Center this text </p>
CSS:
p {
text-align: center;
}
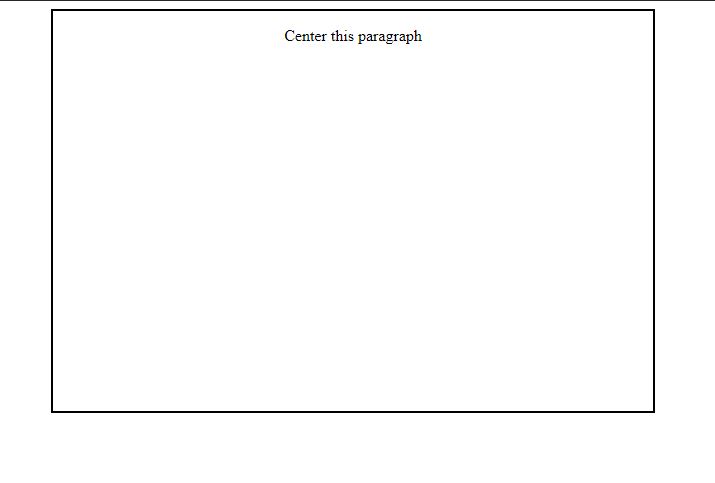
2. Line-height
If you want to center text horizontally, you can use the line-height rule and set the div line height equal to width of that div.
HTML:
<div class="div">
<p class="paragraph"> Center this paragraph </p>
</div>
CSS:
.div {
width: 600px;
height: 400px;
border: 2px solid black;
line-height: 400px;
}
.paragraph {
text-align: center;
}
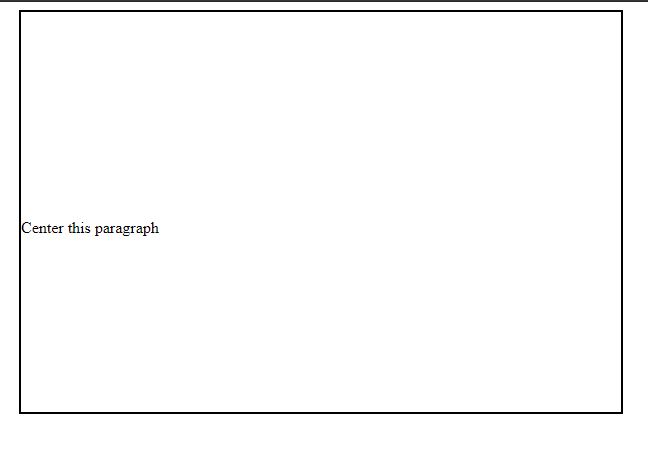
3. Display: table
This technique allows you to add content to an HTML element and center it both horizontally and vertically, without worrying about height or width.
You just need to set display: table in the parent element and display: table-cell in the child element, and then set the vertical and horizontal alignment of the text:
HTML:
<div class="div">
<p class="paragraph"> Center this paragraph </p>
</div>
CSS:
.div {
width: 600px;
height: 400px;
border: 2px solid black;
display: table;
}
.paragraph {
display: table-cell;
text-align: center;
vertical-align: middle;
}
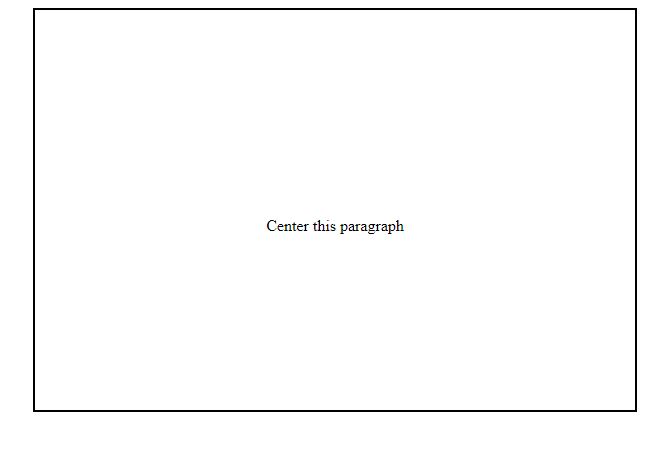